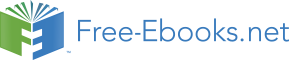

return_type function_name(type(1) argument(1),....,type(n) argument(n))
Syntax of function declaration and declarator are almost same except, there is no semicolon at the end of declarator and function declarator is followed by function body.
In above example, int add(int a,int b) in line 12 is a function declarator.
2. Function body
Function declarator is followed by body of function which is composed of statements.
Passing arguments to functions
In programming, argument/parameter is a piece of data(constant or variable) passed from a program to the function.
In above example two variable, num1 and num2 are passed to function during function call and these arguments are accepted by arguments a and b in function definition.
Arguments that are passed in function call and arguments that are accepted in function definition should have same data type. For example:
If argument num1 was of int type and num2 was of float type then, argument variable a should be of type int and b should be of type float,i.e., type of argument during function call and function definition should be same.
A function can be called with or without an argument.
Return Statement
Return statement is used for returning a value from function definition to calling function.
Syntax of return statement
return (expression);
OR
return;
For example:
return;
return a;
return (a+b);
In above example, value of variable add in add() function is returned and that value is stored in variable sum in main() function. The data type of expression in return statement should also match the return type of function.
Types of User-defined Functions in C Programming
For better understanding of arguments and return in functions, user-defined functions can be categorised as:
1. Function with no arguments and no return value
2. Function with no arguments and return value
3. Function with arguments but no return value
4. Function with arguments and return value.
Let's take an example to find whether a number is prime or not using above 4 cateogories of user defined functions.
Function with no arguments and no return value.
/*C program to check whether a number entered by user is prime or not using function with
no arguments and no return value*/
#include <stdio.h>
void prime();
int main(){
prime(); //No argument is passed to prime().
return 0;
}
void prime(){
/* There is no return value to calling function main(). Hence, return type of prime() is
void */
int num,i,flag=0;
printf("Enter positive integer enter to check:\n");
scanf("%d",&num);
for(i=2;i<=num/2;++i){
if(num%i==0){
flag=1;
}
}
if (flag==1)
printf("%d is not prime",num);
else
printf("%d is prime",num);
}
Function prime() is used for asking user a input, check for whether it is prime of not and display it accordingly. No argument is passed and returned form prime() function.
Function with no arguments but return value
/*C program to check whether a number entered by user is prime or not using function with
no arguments but having return value */
#include <stdio.h>
int input();
int main(){
int num,i,flag;
num=input(); /* No argument is passed to input() */
for(i=2,flag=i;i<=num/2;++i,flag=i){
if(num%i==0){
printf("%d is not prime",num);
++flag;
break;
}
}
if(flag==i)
printf("%d is prime",num);
return 0;
}
int input(){ /* Integer value is returned from input() to calling function */
int n;
printf("Enter positive enter to check:\n");
scanf("%d",&n);
return n;
}
There is no argument passed to input() function But, the value of n is returned from input() to main() function.
Function with arguments and no return value
/*Program to check whether a number entered by user is prime or not using function with
arguments and no return value */
#include <stdio.h>
void check_display(int n);
int main(){
int num;
printf("Enter positive enter to check:\n");
scanf("%d",&num);
check_display(num); /* Argument num is passed to function. */
return 0;
}
void check_display(int n){
/* There is no return value to calling function. Hence, return type of function is void.
*/
int i,flag;
for(i=2,flag=i;i<=n/2;++i,flag=i){
if(n%i==0){
printf("%d is not prime",n);
++flag;
break;
}
}
if(flag==i)
printf("%d is prime",n);
}
Here, check_display() function is used for check whether it is prime or not and display it accordingly. Here, argument is passed to user-defined function but, value is not returned from it to calling function.
Function with argument and a return value
/* Program to check whether a number entered by user is prime or not using function with
argument and return value */
#include <stdio.h>
int check(int n);
int main(){
int num,num_check=0;
printf("Enter positive enter to check:\n");
scanf("%d",&num);
num_check=check(num); /* Argument num is passed to check() function. */
if(num_check==1)
printf("%d in not prime",num);
else
printf("%d is prime",num);
return 0;
}
int check(int n){
/* Integer value is returned from function check() */
int i;
for(i=2;i<=n/2;++i){
if(n%i==0)
return 1;
}
return 0;
}
Here, check() function is used for checking whether a number is prime or not. In this program, input from user is passed to
function check() and integer value is returned from it. If input the number is prime, 0 is returned and if number is not prime, 1 is returned.
C Programming Recursion
A function that calls itself is known as recursive function and the process of calling function itself is known as recursion in C
programming.
Example of recursion in C programming
Write a C program to find sum of first n natural numbers using recursion. Note: Positive integers are known as natural
number i.e. 1, 2, 3....n
#include <stdio.h>
int sum(int n);
int main(){
int num,add;
printf("Enter a positive integer:\n");
scanf("%d",&num);
add=sum(num);
printf("sum=%d",add);
}
int sum(int n){
if(n==0)
return n;
else
return n+sum(n-1); /*self call to function sum() */
}