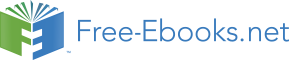

5
15
In, this simple C program, sum() function is invoked from the same function. If n is not equal to 0 then, the function calls itself passing argument 1 less than the previous argument it was called with. Suppose, n is 5 initially. Then, during next function calls, 4 is passed to function and the value of argument decreases by 1 in each recursive call. When, n becomes equal to 0, the value of n is returned which is the sum numbers from 5 to 1.
For better visualization of recursion in this example:
sum(5)
=5+sum(4)
=5+4+sum(3)
=5+4+3+sum(2)
=5+4+3+2+sum(1)
=5+4+3+2+1+sum(0)
=5+4+3+2+1+0
=5+4+3+2+1
=5+4+3+3
=5+4+6
=5+10
=15
Every recursive function must be provided with a way to end the recursion. In this example when, n is equal to 0, there is no recursive call and recursion ends.
Advantages and Disadvantages of Recursion
Recursion is more elegant and requires few variables which make program clean. Recursion can be used to replace complex nesting code by dividing the problem into same problem of its sub-type.
In other hand, it is hard to think the logic of a recursive function. It is also difficult to debug the code containing recursion.
C Programming Storage Class
Every variable and function in C programming has two properties: type and storage class. Type refers to the data type of variable whether it is character or integer or floating-point value etc.
There are 4 types of storage class:
1. automatic
2. external
3. static
4. register
Automatic storage class
Keyword for automatic variable
auto
Variables declared inside the function body are automatic by default. These variable are also known as local variables as they are local to the function and doesn't have meaning outside that function
Since, variable inside a function is automatic by default, keyword auto are rarely used.
External storage class
External variable can be accessed by any function. They are also known as global variables. Variables declared outside every function are external variables.
In case of large program, containing more than one file, if the global variable is declared in file 1 and that variable is used in file 2
then, compiler will show error. To solve this problem, keyword extern is used in file 2 to indicate that, the variable specified is global variable and declared in another file.
Example to demonstrate working of external variable
#include
void Check();
int a=5;
/* a is global variable because it is outside every function */
int main(){
a+=4;
Check();
return 0;
}
void Check(){
++a;
/* ----- Variable a is not declared in this function but, works in any function as they
are global variable ------- */
printf("a=%d\n",a);
}