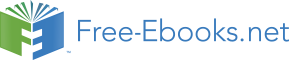

0 5 10
During first function call, it will display 0. Then, during second function call, variable c will not be initialized to 0 again, as it is static variable. So, 5 is displayed in second function call and 10 in third call.
If variable c had been automatic variable, the output would have been:
0 0 0
C Programming Function Examples
This page contains examples and source code on how to work with user-defined function. To understand all the program on this page, you should have knowledge of following function topics:
1. User-Defined Function
2. User-Defined Function Types
3. Storage class( Specially, local variables )
4. Recursion
C Function Examples (solve yourself)
C Programming Examples And Source Code
C Program to Display Prime Numbers Between Intervals by Making Function
C Program to Check Prime and Armstrong Number by Making Function
C program to Check Whether a Number can be Express as Sum of Two Prime
Numbers
C program to Find Sum of Natural Numbers using Recursion.
C program to Calculate Factorial of a Number Using Recursion
C Program to Find H.C.F Using Recursion
C program to Reverse a Sentence Using Recursion
C program to Calculate the Power of a Number Using Recursion
C Programming Examples And Source Code
C Program to Convert Binary Number to Decimal and Decimal to Binary
C Program to Convert Octal Number to Decimal and Decimal to Octal
C Program to Convert Binary Number to Octal and Octal to Binary
C Programming Arrays
In C programming, one of the frequently arising problem is to handle similar types of data. For example: If the user want to store marks of 100 students. This can be done by creating 100 variable individually but, this process is rather tedious and impracticable.
These type of problem can be handled in C programming using arrays.
An array is a sequence of data item of homogeneous value(same type).
Arrays are of two types:
1. One-dimensional arrays
2. Multidimensional arrays( will be discussed in next chapter )
Declaration of one-dimensional array
data_type array_name[array_size];
For example:
int age[5];
Here, the name of array is age. The size of array is 5,i.e., there are 5 items(elements) of array age. All element in an array are of the same type (int, in this case).
Array elements
Size of array defines the number of elements in an array. Each element of array can be accessed and used by user according to the need of program. For example:
int age[5];
Note that, the first element is numbered 0 and so on.
Here, the size of array age is 5 times the size of int because there are 5 elements.
Suppose, the starting addres of age[0] is 2120d and the size of int be 4 bytes. Then, the next address (address of a[1]) will be 2124d, address of a[2] will be 2128d and so on.
Initialization of one-dimensional array:
Arrays can be initialized at declaration time in this source code as:
int age[5]={2,4,34,3,4};
It is not necessary to define the size of arrays during initialization.
int age[]={2,4,34,3,4};
In this case, the compiler determines the size of array by calculating the number of elements of an array.
Accessing array elements
In C programming, arrays can be accessed and treated like variables in C.
For example:
scanf("%d",&age[2]);
/* statement to insert value in the third element of array age[]. */
scanf("%d",&age[i]);
/* Statement to insert value in (i+1)th element of array age[]. */
/* Because, the first element of array is age[0], second is age[1], ith is age[i-1] and
(i+1)th is age[i]. */
printf("%d",age[0]);
/* statement to print first element of an array. */
printf("%d",age[i]);
/* statement to print (i+1)th element of an array. */
Example of array in C programming
/* C program to find the sum marks of n students using arrays */
#include <stdio.h>
int main(){
int marks[10],i,n,sum=0;
printf("Enter number of students: ");
scanf("%d",&n);
for(i=0;i<n;++i){
printf("Enter marks of student%d: ",i+1);
scanf("%d",&marks[i]);
sum+=marks[i];
}
printf("Sum= %d",sum);
return 0;
}