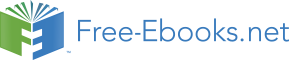

Enter marks of student1: 12
Enter marks of student2: 31
Enter marks of student3: 2
sum=45
Important thing to remember in C arrays
Suppose, you declared the array of 10 students. For example: arr[10]. You can use array members from arr[0] to arr[9]. But, what if you want to use element arr[10], arr[13] etc. Compiler may not show error using these elements but, may cause fatal error during program execution.
C Programming Multidimensional Arrays
C programming language allows to create arrays of arrays known as multidimensional arrays. For example:
float a[2][6];
Here, a is an array of two dimension, which is an example of multidimensional array. This array has 2 rows and 6 columns
For better understanding of multidimensional arrays, array elements of above example can be thinked of as below:
Initialization of Multidimensional Arrays
In C, multidimensional arrays can be initialized in different number of ways.
int c[2][3]={{1,3,0}, {-1,5,9}};
OR
int c[][3]={{1,3,0}, {-1,5,9}};
OR
int c[2][3]={1,3,0,-1,5,9};
Initialization Of three-dimensional Array
double cprogram[3][2][4]={
{{-0.1, 0.22, 0.3, 4.3}, {2.3, 4.7, -0.9, 2}},
{{0.9, 3.6, 4.5, 4}, {1.2, 2.4, 0.22, -1}},
{{8.2, 3.12, 34.2, 0.1}, {2.1, 3.2, 4.3, -2.0}}
};
Suppose there is a multidimensional array arr[i][j][k][m]. Then this array can hold i*j*k*m numbers of data.
Similarly, the array of any dimension can be initialized in C programming.
Example of Multidimensional Array In C
Write a C program to find sum of two matrix of order 2*2 using multidimensional arrays where, elements of matrix are