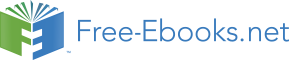

Create a Macro
On the Tools menu, point to Macro, and then click Macros.
NOTE: Macro names must begin with a letter and can contain up to 80 characters. Visual Basic for Applications keywords are invalid names for macros. The name cannot contain any spaces. Programmers typically use an underscore character(_) to separate words.
If you type an invalid macro name, you receive a message similar to the following <macro name> is not a valid name for a macroAdd Code to a New Macro
You are now looking at a flashing insertion point within the Code window. The Code window is where you actually type Visual Basic commands. A recorded macro can also be viewed in the code window. For the most part, the Code window acts like a typical text editor, enabling you to cut, copy, and paste text.
However, there are some differences that make it easier for you to create macros. The important differences are detailed below.Dim MySlide As Slide
When you were typing in the code, you probably noticed some interesting things happen. After you hit the spacebar following the word as, a drop down list of the available data types appeared on your screen. This is just one of the ways the Visual Basic Editor makes programming a little easier.
What does this code do?
Dim Indicates to the Visual Basic Editor you are about to declare a variable. There are several other methods available to
declare variables, but this article discusses only the Dim method.
This code adds a new slide to the active presentation. The slide created uses the Title Only AutoLayout. Lets take a closer look at this line of code.
Set MySlide Assigns an object reference to a variable or property. Using Set makes it easier to refer to that same object later in your code.
Sub YourMacro ()
'
' Macro created 1/7/97 by You
'
Dim MySlide As Slide
Set MySlide = ActivePresentation.Slides.Add(1, ppLayoutTitle)
Run the Macro
There are several methods to run a macro. Only one method is described in this article. On the File menu, click Close and Return to Microsoft PowerPoint.
View the Macro Code
To view the source code of a specific macro, follow these steps: On the Tools menu, point to Macro, and then click Macros.
Add Some More Code
You are now ready to add the rest of the commands to complete the macro.
With ActiveWindow.Selection.SlideRange
.FollowMasterBackground = msoFalse
.Background.Fill.PresetTextured msoTextureRecycledPaper
These commands tell PowerPoint that this particular slide does not follow the master, and then set the background preset texture to the recycled paper.
The With statement allows you to group commands that have common references. Using With to group multiple commands can improve the performance of the macro as well as saving you a lot of typing.
You can see the advantage of using With statements: less typing and faster code. The main disadvantage of the With statement is that it sometimes makes the code more difficult to read, especially if you nest a With within another With statement.
Add the next line of code to your macro:With ActivePresentation.Slides.Range.SlideShowTransition .AdvanceTime = 5
.EntryEffect = ppEffectCheckerboardAcross
The Complete Macro Code
Sub YourMacro()
'
' Macro created <Date> by <You> '
Dim MySlide As Slide
' Apply a preset texture to the slide.
With ActiveWindow.Selection.SlideRange
.FollowMasterBackground = msoFalse
With ActivePresentation.Slides.Range.SlideShowTransition .AdvanceTime = 5
.EntryEffect = ppEffectCheckerboardAcross
End With
' Start the slide show.
ActivePresentation.SlideShowSettings.Run
Project the presentation on an LCD Projector Export to Overheads
Export to 35mm Slides
Even though the instructions given in this book are relatively simple, every computer-projector combination is different and it is very common for something to go wrong. Hence, you need to try out in advance if possible by having a demo projection, and still leave yourself plenty of time to set up the final show.
Advance preparationsIf your presentation is having any media with sound, you will need a second cable to link your laptop’s headphone/speaker jack to the audio input on the projector. This cable looks like a walkman headphone jack on both ends. If you have those cables, you can connect them directly, but if not, they should be fairly cheap to buy at an electronics store. If you check your computer speakers, there might be one running between them which you can detach. As a last resort, you could bring in your computer speakers and hook those up to your computer instead
Find out in advance which key combination will get your computer screen to display from the projector. Unfortunately it is not the same for all computers. It is usually Fn + some F-key. On Dell laptops it is Fn + F8. On Toshiba laptops it is Fn + F5. On at least some IBM laptops it is Fn + F7. If you’re lucky, the correct F-key on your laptop will say “CRT/LCD” or have a tiny icon of a computer and screen. Even in some other laptops you will find a picture of projector in the related Function Key. If you have no clue what works, you can try pressing Fn + each Fkey without the projector connected. When your screen temporarily flashes black, and/or if some icons of monitors appear, you have probably found the right combination. If your screen stays black, that’s still a good sign – just keep pressing the same two keys until your display returns.
Setting up the presentationFor setting up the presentation, start with both projector and laptop off. (If your computer is already turned on you can try following these instructions anyway – sometimes it works).
Plug in the projector.
Attach your laptop to the projector with the main cable, and the audio cable if necessary.
Turn on the projector. It may take a while to warm up. You will not see your slideshow yet, but you should see a blue screen projected onto the wall. If not, check to make sure the power is on and the lens cap is off. Turn on the computer. Wait for it to boot up, and then press the required keys for projector display (Fn + F8 on a Dell laptop). Your computer screen might go black for a few seconds, but then you should see it displayed on the wall. If this works but your laptop screen remains black, press Fn + F8 (or whatever keys you pressed before) one more time.
If you are using audio, play a sound file and check the volume. You should be able to turn the projector volume up fairly high, even though the sound quality is not great. Turn the volume up on your computer as well, if necessary. If you still don’t hear anything at all, make sure the mute function is off on both projector and computer, and that the audio input cord is pushed all the way into projector and laptop ports.
Open PowerPoint and your slideshow file. Press F5 to start your slideshow.
Yes, it is possible to get your slideshow to display on the wall and your lecture notes (or whatever you want) to display on your laptop screen. You need to use dual monitor configuration for this. Like setting up a projector, it is often a lot more complicated than it should be, and it varies by computer. You can find instructions in the PowerPoint help files if you like, or through Google.
• Try other key combinations. Press all the F-keys in turn. Press the Fn-key with each F-key. Something has to work!
• Make sure the resolution of your laptop matches that of the projector:
a) Look on the projector and see if you can find the resolution written on it. It is probably either 1024x768 or 800x600. If you can’t find it, try these instructions anyway.
b) On your laptop, go to Control Panel Display, then click on the Settings tab.
c) Under Screen Resolution, move the slider to the correct resolution. If you don’t know, try 1024x768 pixels. Click on Apply. If you still can’t get your screen to display, try 800x600 pixels. Keep trying resolutions until one works.
• Sometimes there are different input sources on the projector. Find the input button and cycle through these.• Open your PowerPoint file, then press F5 to start your show.
• Move through your slideshow either by clicking on the mouse or by using the up and down arrows on your keyboard. (Some projectors also come with a remote control). A bunch of fancy commands for jumping around in your slideshow are available at: http://office.microsoft.com/en-gb/assistance/HP051953031033.aspx
• If you need to go backwards, press the up arrow.• If you move your mouse pointer over the bottom left-hand corner of the screen, you will see a menu with several advanced options. If you click on the pen icon, you can get a “pen” that allows you to draw on your slides (in non-permanent ink).
• To leave the slideshow at any time, hit ESC.You can print transparencies directly from the presentation without printing out the paper copies and running transparencies from the paper copies through your copier. Just load the transparencies into your printer and print one or two slides to make sure everything is working correctly. Then do the remaining slides.
If you have a color printer you can print color transparencies directly, too.If you eventually want to output your PowerPoint presentation to 35mm slides, you should change the page setup before you begin entering data. 35mm slides are slightly wider than slides used in presentations. Changing the page setup after you've completed your presentation might radically change the formatting.
So, the instant you begin creating a 35mm slide show, go into File | Page Setup | Slides Sized For | 35mm slides. Now you have the correct aspect ratio for 35mm slides.By default, PowerPoint automatically checks the presentation for consistency and style, and marks problems on a slide with a light bulb. You can fix or ignore these errors and also change the elements that PowerPoint checks for. The light bulb is not available if you have turned off the Office Assistant. To turn on the Assistant, click Show the Office Assistant on the Help menu.
• Open the presentation you want to check for style and consistency.• Click the light bulb and then click the option you want in the list. To follow up with Meeting Minder and Action Items
Participants in an online meeting can also use the Meeting Minder dialog box or the Speaker Notes dialog box to take notes. The notes are visible to all participants. These features are available only when the presentation is in Slide Show view.
• To add notes or meeting minutes, right-click the slide and then click Meeting Minder or SpeakerNotes.
• Click in the box and then type the notes or minutes.
• To add an action item, right-click the slide, click Meeting Minder and then click the Action Items
tab.
• Click in the box, type the information for the first action item, and then click Add.
• Repeat step 2 for every action item and then click OK. The action items appear on a new slide at
Master slides and custom templates
If you use PowerPoint on a regular basis for presenting related materials, you may want to use master slides and create a custom template. With the master slides and custom template, all of your presentations will have a consistent look and feel, and you won't have to customize each presentation layout separately.
Customizing your slides using the slide masterThe slide master allows you to customize the look of each slide and ensure consistency across your presentation. In the slide master, you can change fonts, bullets, and header and footer information.
To have art or text—for example, a company name or logo—appear on every slide, put it on the slide master. Objects appear on slides in the same location as they do on the slide master. To add the same text to every slide, add the text to the slide master by clicking Text Box on the Drawing toolbar—do not type in the text placeholders. The look of text you've added with Text Box is not governed by the slide master.
To change master text and title stylesThe date, footer, and number areas can all be changed in the same way. Experiment to see which combinations you like best. Remember that the slide master changes every slide in your presentation
• On the View menu, point to Master and then click Slide Master.
• Click anywhere in the Click to edit Master title styles text block.
• On the Standard toolbar, click the Font down arrow and then click Arial.
• On the Standard toolbar, click the Font Size down arrow and then click 40.
• Right-mouse click anywhere on Click to edit Master text styles and then click Bullet.
• In the Bullets and Numbering list box and then click Pictures.
• Click the multi-color block in the first row of the third column and then click Insert Clip.
Resist the temptation to drag & drop PowerPoint files to a memory Stick, unless you are absolutely sure the presentation has no link to any other file (audio, video, excel sheet etc.). If you copied your linked files into the folder with your slideshow as you were creating it, you can afford to drag & drop the presentation into the memory stick. If you have a newer version of PowerPoint installed in your PC/Laptop, various tools are available, which makes copying even easier.
PowerPoint 2003PowerPoint 2003 allows transfer of files in few clicks. Go to >File – Package for CD. If you are burning to a CD, insert the CD & click on Copy to CD. If you are copying to a memory stick, insert it, Choose copy to folder & locate the appropriate drive. All of your linked files should automatically be included, regardless of whether or not you copied them all into the same folder. You also get a copy of PowerPoint viewer, which allows the Show to play even if the target computer doesn’t have PowerPoint installed.
PowerPoint 2002In PowerPoint 2002, you should be able to use the command, File – Pack & Go, but make sure to check the boxes marked Include Linked Files and Embed True Type fonts. This process also gives you the option of downloading the PowerPoint Viewer if you don’t already have it.
PowerPoint 2002 zips your files; hence you need to unzip them on the new computer.In all older versions of PowerPoint, you will have to rely on copying the entire folder method. If you don’t have PowerPoint in the target machine, you can download the PowerPoint Viewer & install it in the target machine. What I would suggest to all PowerPoint users is to have a copy of PowerPoint Viewer with them all the time. You can also carry a copy of PowerPoint Viewer in the memory stick.
Working with Text
Identifying Toolbars
Page Setup
Creating Action Buttons
Adding Objects to Slides Using the Drawing Toolbar Advanced Drawing Techniques
Combining Presentations
Creating Original Artworks
Builds & Transitions
Setting Animation
Saving a slide as a graphic file.
Organization Charts
Showing Off your Presentation at a KIOSK Annotating Slides
Style Checker
This advanced module of PowerPoint is intended for those who have already mastered the basics of PowerPoint. Working with Text Reminders about Fonts
It is preferable to use a sans serif font type in a presentation because a font without serifs is easier to read on a screen. Serif fonts like Times New Roman or Palatino or Courier tend to drag the viewer's eye back to the line. The serifs themselves do not translate well into on-screen presentations and are best kept for print-based book chapters and articles.
The best presentation fonts are Arial, Helvetica and Tahoma.Text and objects can be placed right up to the edges of a slide but for accuracy of placement, use guides. To get additional information on guides see below. To align objects, select the object or objects you want to align from the Drawing toolbar, click Draw, then point to Align or Distribute, following it with the alignment you need. Possibilities include:
Align Left
Align Right Align Center Align Middle Align Bottom and so on...
Use Insert | Picture | WordArt or from the Drawing Toolbar, select the WordArt icon. Select the WordArt format you want. Click OK. Type in the text (keep it short). Next select the Font and Font Size, bolding or italics and click OK. If the text looks the way you want it to look on the slide, grab the handles at the corner of the object and tug it carefully into the size you want. Reposition the object by clicking in the middle of the image; hold down the left mouse button and drag it into place. If you want to make any changes, double-click on the middle of the object and PowerPoint will bring up the WordArt window.
Be judicious in your use of WordArt. A little goes a long way.To shadow all slide titles, go into the Slide View master, click the dashed line in the title box and when you see the hatched line, click the Shadow Button. Now all titles will be shadowed. This is not an affect I particularly care for so I rarely use it - but many do.
Creating Mirrored TextFor some reason you might wish to have a word or phrase appear as its mirror image. To do this, use WordArt to create the text. Click on the text to select it. Then do File | Copy, then File Paste. Move the second copy directly underneath the first. If the second image is not selected, click on it. That will select it. From Draw select Rotate or Flip, then Flip Horizontal. This does give you a different view of things.
Guides and Rulers.Use guides to move and place objects exactly where you want them. Guides can be used to group objects, or to rotate objects in relations to the edges of a portion of the document. If you are a good designer, you can use the guides to stack objects. Stacking objects involves drawing objects and placing them on top of each other. Stacked objects can be brought forward or sent backward depending on the commands you use.
To view the Rulers or Guides, select Slide view, then from the View menu, select Guides and then select View | Rulers
To add a guide, hold down Ctrl, grab and drag an existing guide, for example, the guide dividing the slide in half vertically. To delete a guide, drag it off the slide. You may hide the guides without deleting them, by selecting Guides on th
Reads:
108
Pages:
176
Published:
Apr 2023
"No Filter, No Problem" by Famium is your ultimate guide to creating a visually stunning, engaging Instagram presence. Packed with insider secrets and practic...
Formats: PDF, Epub, Kindle, TXT