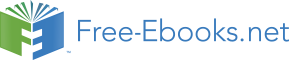

10. Assigning values
In the last chapter we saw the keyword
value
as well as the use of the assign statement, which is accomplished by the equal sign. Before proceeding, let us look at some examples of both these concepts since there are two different ways of accomplishing the same result. The
value
keyword allows us to give a variable some initial value. Thus
define x integer(5) value 7
results in the field
x
being equal to 7, while
define y decimal(2.2) value 3.1
results in the field
y
being 03.10. In the first case
x
consists of five positions with the last being 7, preceded by four leading zeroes. The values 7 and 00007 are equal. The computer will store that number with the leading zeroes. In a similar manner, 3.1 = 03.10, but the computer will actually have 0310 for this field without the decimal point, and it is understood to be there. In our programs we don’t need to specify leading zeroes if fields are defined integer or decimal. For character fields we have to supply leading zeroes, if they exist. Consider the following:
define soc-sec-no character(9) value “45169123”
This will result in that variable being the eight characters above followed by a space which is in the ninth position. As of today, that is not a valid social security number. If we really wanted the first position to be a leading zero as in some social security numbers, our value clause would have to be
value “045169123”
rather than
value “45169123”.
Note that this would not be the case if we had defined the variable as an integer since the leading zero would have been assumed.
As we have already seen
define z character(5) value “Page”
results in
z
being Page where the rightmost position is a space. This means that fields that are defined as numeric, whether
integer
or
decimal
will fill the field with leading zeroes where necessary while those defined as
character
will fill the trailing positions with spaces if needed.
This same consideration will apply with assignment statements. Using
soc-sec-no
and
x
as defined above, the statement
x = 3
will result in
x
being 00003 inside the computer, while
soc-sec-no = “111111”
will result in
soc-sec-no
being 111111 , that is, six 1’s followed by three spaces. Note that assignment statements involving
character
fields need the quote marks while those dealing with numeric fields will not use them. We could eliminate the
value
keyword and accomplish the same result by using a single assign statement. All we have to do is utilize a group of assign lines just after the last
define
keyword, one for each
value
occurrence. Since we do have the
value
keyword, we have the option of giving a field a value in one of two ways. One is initialization and the other an assignment statement, which often changes the value.
Since this concept is so important in programming, let us consider a few more examples. Assume the following
define x character(6) value “Handel”
define y character(4)
If the following line is executed,
y = x
x
will still have the value
Handel
but
y
will have the value
Hand,
since the field
y
only has room for 4 characters, it can only accept that many and so truncation will occur. Note that in any assign statement, the variable on the right side of the equal sign will be unchanged. If the first assign is done followed by
x = y
the result will be that
x
will have the value
Hand ,
that is the rightmost two characters will be spaces and
y
will be
Hand,
since that value won’t change from the first assignment.
Now let us consider
define a decimal(6.2) value 82344.57
define b decimal(4.1)