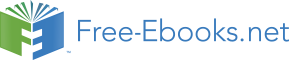

b = a,
a
will be unchanged but
b
will have the value 2344.5. Thus truncation will occur on both the left since the variable
b
only allows for 4 digits to the left of the decimal point as well as on the right since there is only room for a single digit to the right of the decimal point. Note that the number that results in
b
is not rounded. You may never run into this situation exactly but may need something similar in order to truncate a value.
We have seen the following before but since we will be using it over and over, it is worth repeating.
define line-counter integer(2) value 0
loop: line-counter = line-counter + 1
if line-counter > 9
go to end-program
end-if
go to loop
end-program: end
The statement following the
define
will be executed 10 times, that is until
line-counter
is greater than 9. The variable
line-counter
will start out as 0 and
loop: line-counter = line-counter + 1
will result in 1 being added to the field and 1 will now be the new value of
line-counter.
A check on the value of the variable is now done and since
line-counter
is not greater than 9, a branch will be made to the label
loop.
Then
line-counter
will become 2 and the check will be done again and once more a branch is made to the label
loop.
As the program continues to be executed,
line-counter
will become 3, 4, 5, 6, 7, 8, 9 and then 10 and now since
line-counter
is greater than 9, a branch will be taken to the label
end-program
and there is no further action.
This statement at the label
loop
is a way to increment a field, such as we had in our program when we needed to keep track of the lines printed in order to know if a new set of headings was needed. Of course we could increment this field by 2 or 3 or even a negative number if we desired. If our increment statement had been
loop: line-counter = line-counter – 2
note than we would have the following values for
line-counter:
2, 4, 6, 8, and 10. If you think it should be -2, -4, -6, -8, -10 because we are subtracting 2, recall that the variable
line-counter
is defined integer, with no provision for the sign. Thus the first increment will do the subtraction but since the computer has no place for a negative sign, the
line-counter
will be 2 at first. Eventually it will get to 10 and we will branch to the label
end-program.
If we have
define line-counter signed integer(2) value 0
loop: line-counter = line-counter - 2
if line-counter > 9
go to end-program
end-if
go to loop
end-program: end
note what will happen now. The field
line-counter
will be 0 to start and then -2, which is what we want, and then -4, -6, -8 and -10. But it will not stop there as we will be stuck in a loop since
line-counter
is not getting closer to the value 9, it is proceeding in the opposite direction, becoming a smaller negative number. We could change the lines above by initially setting the value of
line-counter
to 10 and changing the decision line to
if line-counter = 0
and now the endless looping will not take place.
Consider the following
define j character(11) value “candlelight”
define k character(6)
define m character(5)
k = j(1:6)
m = j(7:5)
If we look at the variables after the assign statements,
k
will be candle and
m
will be light.
That’s because we’re getting pieces of a string of characters from one variable. The expression
j(1:6)
will result in the characters in the variable
j
starting in position 1 for 6 positions being moved to the variable
k,
so it becomes the value candle. For the variable
m
5 characters starting in position 7 will be moved to
m,
which happens to be the value light. This may come in handy some time and it also means that we could eliminate the
structure
keyword since we could extract any field we want from
account-record
by using an expression like
last-name = account-record(10:18)
which will give us the last name from the record. However, we won’t eliminate the keyword
structure
as it just might save us some time and work. As in many situations, we have some freedom and can use whichever method is more beneficial.