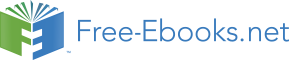

3. Input to and output from a F95 program
We have already seen some examples of outputting information from a program (write) and reading information from the terminal (read). In this section we will look in detail at input and output and explain those strange ‘*’s. To save some writing let’s introduce some jargon: we will call input and output I/O.
3.1 F95 statements for I/O
Input and output to a F95 program are controlled by the read and write statements. The manner in which this is done is controlled by format descriptors, which may be given as character variables or provided in a format statement. For economy of effort we will only outline the latter method, together with the default mechanism.
The form of the I/O statements is as follows:
read(stream, label [, end=end][, err=err]) list
and
write(stream, label) list
where
* here indicates the default value, usually the screen of a terminal session. If stream is a character variable, the result of the write is stored in that variable, and can be manipulated as such within the program.
The precise details of how the output should look are governed by the format definition. This takes the form:
label format (format descriptors)
To access a file for input or output you can use the open statement:
open([unit=]stream, err=escape, action=action, file=name)
There are further possible arguments which should not be needed for this course, but which you may look up in a text book.
Having opened a file, linking it to a stream, and read through it, you can move back to the beginning using:
rewind( stream)
When you have completed I/O to a particular file, you can use the close instruction to close the file and tidy things up:
close( stream)