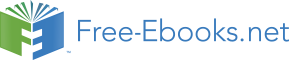

CHAPTER
BYTECODE ANALYSIS
There are several keys ways that you can make your code run faster. Having an understanding of what’s happening in the background can be useful. Python’s dis module lets us disassemble the code to see the underlying bytecode.
We can use dis.dis(fn) to disassemble the bytecode which represents fn. First we’ll import pure_python to bring our module into the namespace:
>>>importpure_python # imports our solver into Python
>>> dis.dis(pure_python.calculate_z_serial_purepython)
....
18 109 LOAD_FAST 2 (z) # load z
112 LOAD_FAST 4 (i) # load i
115 BINARY_SUBSCR # get value in z[i]
116 LOAD_FAST 2 (z) # load z
119 LOAD_FAST 4 (i) # load i
122 BINARY_SUBSCR # get value in z[i]
123 BINARY_MULTIPLY # z[i] * z[i]
124 LOAD_FAST 0 (q) # load z
127 LOAD_FAST 4 (i) # load i
130 BINARY_SUBSCR # get q[i]
131 BINARY_ADD # add q[i] to last multiply
132 LOAD_FAST 2 (z) # load z
135 LOAD_FAST 4 (i) # load i
138 STORE_SUBSCR # store result in z[i]
19 139 LOAD_GLOBAL 2 (abs) # load abs function
142 LOAD_FAST 2 (z) # load z
145 LOAD_FAST 4 (i) # load i
148 BINARY_SUBSCR # get z[i]
149 CALL_FUNCTION 1 # call abs
152 LOAD_CONST 6 (2.0) # load 2.0
155 COMPARE_OP 4 (>) # compare result of abs with 2.0
158 POP_JUMP_IF_FALSE 103 # jump depending on result
...
Above we’re looking at lines 18 and 19. The right column shows the operations with my annotations. You can see that we load z and i onto the stack a lot of times.
Pragmatically you won’t optimise your code by using the dis module but it does help to have an understanding of what’s going on under the bonnet.