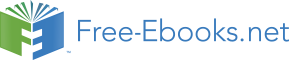

Interpolation with MATLAB.
Linear interpolation is one of the most common techniques for estimating values between two given data points. For example, when using steam tables, we often have to carry out interpolations. With this technique, we assume that the function between the two points is linear. MATLAB has a built-in interpolation function.
interp1
Function Give an x-y table, y_new = interp1(x,y,x_new)
interpolates to find y_new. Consider the following examples:
To demonstrate how the interp1
function works, let us create an x-y table with the following commands;
x = 0:5; y = [0,20,60,68,77,110];
To tabulate the output, we can use
[x',y']
The result is
ans = 0 0 1 20 2 60 3 68 4 77 5 110
Suppose we want to find the corresponding value for 1.5 or interpolate for 1.5. Using y_new = interp1(x,Y,x_new)
syntax, let us type in:
y_new=interp1(x,y,1.5) y_new = 40
The table we created above has only 6 elements in it and suppose we need a more detailed table. In order to do that, instead of a single new x value, we can define an array of new x values, the interp1
function returns an array of new y values:
new_x = 0:0.2:5; new_y = interp1(x,y,new_x);
Let's display this table
[new_x',new_y']
The result is
ans = 0 0 0.2000 4.0000 0.4000 8.0000 0.6000 12.0000 0.8000 16.0000 1.0000 20.0000 1.2000 28.0000 1.4000 36.0000 1.6000 44.0000 1.8000 52.0000 2.0000 60.0000 2.2000 61.6000 2.4000 63.2000 2.6000 64.8000 2.8000 66.4000 3.0000 68.0000 3.2000 69.8000 3.4000 71.6000 3.6000 73.4000 3.8000 75.2000 4.0000 77.0000 4.2000 83.6000 4.4000 90.2000 4.6000 96.8000 4.8000 103.4000 5.0000 110.0000
Using the table below, find the internal energy of steam at 215 ˚C and the temperature if the internal energy is 2600 kJ/kg (use linear interpolation).
Temperature [C] | Internal Energy [kJ/kg] |
---|---|
100 | 2506.7 |
150 | 2582.8 |
200 | 2658.1 |
250 | 2733.7 |
300 | 2810.4 |
400 | 2967.9 |
500 | 3131.6 |
First let us enter the temperature and energy values
temperature = [100, 150, 200, 250, 300, 400, 500]; energy = [2506.7, 2582.8, 2658.1, 2733.7, 2810.4, 2967.9, 3131.6]; [temperature',energy']
returns
ans = 1.0e+003 * 0.1000 2.5067 0.1500 2.5828 0.2000 2.6581 0.2500 2.7337 0.3000 2.8104 0.4000 2.9679 0.5000 3.1316
issue the following for the first question:
new_energy = interp1(temperature,energy,215)
returns
new_energy = 2.6808e+003
Now, type in the following for the second question:
new_temperature = interp1(energy,temperature,2600)
returns
new_temperature = 161.4210
Linear interpolation is a technique for estimating values between two given data points,
Problems involving steam tables often require interpolated data,
MATLAB has a built-in interpolation function.
Problem Set for Interpolation with MATLAB
Determine the saturation temperature, specific liquid enthalpy, specific enthalpy of evaporation and specific enthalpy of dry steam at a pressure of 2.04 MPa.
Pressure [MN/m2] | Saturation Temperature [C] | hf [kJ/kg] | hfg [kJ/kg] | hg [kJ/kg] |
---|---|---|---|---|
2.1 | 214.9 | 920.0 | 1878.2 | 2798.2 |
2.0 | 212.4 | 908.6 | 1888.6 | 2797.2 |
>> pressure=[2.1 2.0]; >> sat_temp=[214.9 212.4]; >> h_f=[920 908.6]; >> h_fg=[1878.2 1888.6]; >> h_g=[2798.2 2797.2]; >> sat_temp_new=interp1(pressure,sat_temp,2.04) sat_temp_new = 213.4000 >> h_f_new=interp1(pressure,h_f,2.04) h_f_new = 913.1600 >> h_fg_new=interp1(pressure,h_fg,2.04) h_fg_new = 1.8844e+003 >> h_g_new=interp1(pressure,h_g,2.04) h_g_new = 2.7976e+003
The following table gives data for the specific heat as it changes with temperature for a perfect gas. (Data available for download). [8]
Temperature [F] | Specific Heat [BTU/lbmF] |
---|---|
25 | 0.118 |
50 | 0.120 |
75 | 0.123 |
100 | 0.125 |
125 | 0.128 |
150 | 0.131 |
Using interp1 function calculate the specific heat for 30 F, 70 F and 145 F.
>> temperature=[25;50;75;100;125;150] temperature = 25 50 75 100 125 150 >> specific_heat=[.118;.120;.123;.125;.128;.131] specific_heat = 0.1180 0.1200 0.1230 0.1250 0.1280 0.1310 >> specific_heatAt30=interp1(temperature,specific_heat,30) specific_heatAt30 = 0.1184 >> specific_heatAt70=interp1(temperature,specific_heat,70) specific_heatAt70 = 0.1224 >> specific_heatAt145=interp1(temperature,specific_heat,145) specific_heatAt145 = 0.1304
For the problem above, create a more detailed table in which temperature varies between 25 and 150 with 5 F increments and corresponding specific heat values.
>> new_temperature=25:5:150; >> new_specific_heat=interp1(temperature,specific_heat,new_temperature); >> [new_temperature',new_specific_heat'] ans = 25.0000 0.1180 30.0000 0.1184 35.0000 0.1188 40.0000 0.1192 45.0000 0.1196 50.0000 0.1200 55.0000 0.1206 60.0000 0.1212 65.0000 0.1218 70.0000 0.1224 75.0000 0.1230 80.0000 0.1234 85.0000 0.1238 90.0000 0.1242 95.0000 0.1246 100.0000 0.1250 105.0000 0.1256 110.0000 0.1262 115.0000 0.1268 120.0000 0.1274 125.0000 0.1280 130.0000 0.1286 135.0000 0.1292 140.0000 0.1298 145.0000 0.1304 150.0000 0.1310
During a 12-hour shift a fuel tank has varying levels due to consumption and transfer pump automatically cutting in and out to maintain a safe fuel level. The following table of fuel tank level versus time (Data available for download) is missing readings for 5 and 9 AM. Using linear interpolation, estimate the fuel level at those times.
Time [hours, AM] | Tank level [m] |
---|---|
1:00 | 1.5 |
2:00 | 1.7 |
3:00 | 2.3 |
4:00 | 2.9 |
5:00 | ? |
6:00 | 2.6 |
7:00 | 2.5 |
8:00 | 2.3 |
9:00 | ? |
10:00 | 2.0 |
11:00 | 1.8 |
12:00 | 1.3 |
>> time=[1 2 3 4 6 7 8 10 11 12]; >> tank_level=[1.5 1.7 2.3 2.9 2.6 2.5 2.3 2.0 1.8 1.3]; >> tank_level_at_5=interp1(time,tank_level,5) tank_level_at_5 = 2.7500 >> tank_level_at_9=interp1(time,tank_level,9) tank_level_at_9 = 2.1500