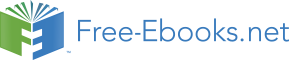

Essential skills to use MATLAB effectively.
Learning a new skill, especially a computer program in this case, can be overwhelming. However, if we build on what we already know, the process can be handled rather effectively. In the preceding chapter we learned about MATLAB Graphical User Interface (GUI) and how to get help. Knowing the GUI, we will use basic math skills in MATLAB to solve linear equations and find roots of polynomials in this chapter.
The evaluation of expressions is accomplished with arithmetic operators as we use them in scientific calculators. Note the addtional operators shown in the table below:
Operator | Name | Description |
---|---|---|
+ | Plus | Addition |
- | Minus | Subtraction |
* | Asterisk | Multiplication |
/ | Forward Slash | Division |
\ | Back Slash | Left Matrix Division |
^ | Caret | Power |
.* | Dot Asterisk | Array multiplication (element-wise) |
./ | Dot Slash | Right array divide (element-wise) |
.\ | Dot Back Slash | Left array divide (element-wise) |
.^ | Dot Caret | Array power (element-wise) |
The backslash operator is used to solve linear systems of equations, see the section called “Linear Equations”.
Matrix is a rectangular array of numbers and formed by rows and columns. For example
. In this example A consists of 4 rows and 4 columns and therefore is a 4x4 matrix. (see Wikipedia).
Row vector is a special matrix that contains only one row. In other words, a row vector is a 1xn matrix where n is the number of elements in the row vector.
Column vector is also a special matrix. As the term implies, it contains only one column. A column vector is an nx1 matrix where n is the number of elements in the column vector.
Array operations refer to element-wise calculations on the arrays, for example if x is an a by b matrix and y is a c by d matrix then x.*y can be performed only if a=c and b=d. Consider the following example, x consists of 2 rows and 3 columns and therefore it is a 2x3 matrix. Likewise, y has 2 rows and 3 columns and an array operation is possible.
and
then
The following figure illustrates a typical calculation in the Command Window.
MATLAB allows us to build mathematical expressions with any combination of arithmetic operators. The order of operations are set by precedence levels in which MATLAB evaluates an expression from left to right. The precedence rules for MATLAB operators are shown in the list below from the highest precedence level to the lowest.
Parentheses ()
Power (^)
Multiplication (*), right division (/), left division (\)
Addition (+), subtraction (-)
MATLAB has all of the usual mathematical functions found on a scientific calculator including square root, logarithm, and sine.
Typing pi
returns the number 3.1416. To find the sine of pi, type in sin(pi)
and press enter.
The arguments in trigonometric functions are in radians. Multiply degrees by pi/180 to get radians. For example, to calculate sin(90), type in sin(90*pi/180)
.
In MATLAB log
returns the natural logarithm of the value. To find the ln of 10, type in log(10) and press enter, (ans = 2.3026).
MATLAB accepts log10
for common (base 10) logarithm. To find the log of 10, type in log10(10) and press enter, (ans = 1).
Practice the following examples to familiarize yourself with the common mathematical functions. Be sure to read the relevant help
and doc
pages for functions that are not self explanatory.
Calculate the following quantities:
,
50.5−1
for d=2
MATLAB inputs and outputs are as follows:
is entered by typing
2^3/(3^2-1)
(ans = 1)
50.5−1
is entered by typing sqrt(5)-1
(ans = 1.2361)
for d=2 is entered by typing
pi/4*2^2
(ans = 3.1416)
Calculate the following exponential and logarithmic quantities:
ⅇ2
ln(510)
log(105)
MATLAB inputs and outputs are as follows:
exp(2)
(ans =
7.3891)
log((5^10))
(ans =
16.0944)
log10(10^5)
(ans =
5)
Calculate the following trigonometric quantities:
tan(45)
sin(π)+cos(45)
MATLAB inputs and outputs are as follows:
cos(pi/6)
(ans =
0.8660)
tan(45*pi/180)
(ans =
1.0000)
sin(pi)+cos(45*pi/180)
(ans =
0.7071)
format
Function The format
function is used to control how the numeric values are displayed in the Command Window. The short
format is set by default and the numerical results are displayed with 4 digits after the decimal point (see the examples above). The long
format produces 15 digits after the decimal point.
Calculate and display results in
short
and long
formats.
The short
format is set by default:
>> theta=tan(pi/3) theta = 1.7321 >>
And the long
format is turned on by typing format long
:
>> theta=tan(pi/3) theta = 1.7321 >> format long >> theta theta = 1.732050807568877
In MATLAB, a named value is called a variable. MATLAB comes with several predefined variables. For example, the name pi refers to the mathematical quantity π, which is approximately pi ans = 3.1416
MATLAB is case-sensitive, which means it distinguishes between upper- and lowercase letters (e.g. data, DATA and DaTa are three different variables). Command and function names are also case-sensitive. Please note that when you use the command-line help, function names are given in upper-case letters (e.g., CLEAR) only to emphasize them. Do not use upper-case letters when running functions and commands.
Variables in MATLAB are generally represented as matrix quantities. Scalars and vectors are special cases of matrices having size 1x1 (scalar), 1xn (row vector) or nx1 (column vector).
The term scalar as used in linear algebra refers to a real number. Assignment of scalars in MATLAB is easy, type in the variable name followed by = symbol and a number:
a = 1
Elements of a row vector are separated with blanks or commas.
Let's type the following at the command prompt:
b = [1 2 3 4 5]
We can also use the Variable Editor to assign a row vector. In the menu bar, select File > New > Variable. This action will create a variable called unnamed which is displayed in the workspace. By clicking on the title unnamed, we can rename it to something more descriptive. By double-clicking on the variable, we can open the Variable Editor and type in the values into spreadsheet looking table.
Elements of a column vector is ended by a semicolon:
c = [1;2;3;4;5;]
Or by transposing a row vector with the ' operator:
c = [1 2 3 4 5]'
Or by using the Variable Editor:
Matrices are typed in rows first and separated by semicolons to create columns. Consider the examples below:
Let us type in a 2x5 matrix:
d = [2 4 6 8 10; 1 3 5 7 9]